F# Interview Questions and Answers for freshers experienced :-
1. What is F#?
F# is a functional and cross-platform programming language. F# programming language is used for .Net Framework.
2. What are the features of F#?
The features of F# are:
- Net implementation of OCaml
- Provides type inference
- Rich pattern matching constructs
- Interactive scripting and debugging capabilities
- Writing higher order functions
3. Write a program in F# to print “F# Expert” by using printfn?
Example:
printfn “F# Expert”
4. Write a program in F# to print “Interview Questions” by using System.Console?
Example:
System.Console.WriteLine(“Interview Questions”)
5. What are the types of data types used in F#?
The types of data types used in F# are:
- Unit type
- Unit type
- Derived data types
- Primitive data types
6. What are the types of comment in F#?
There are two types of comment in F#:
Single line. Example: // Single Line Comment.
Multiple line. Example:(* Multiple Line Comment.*)
7. What is Tuple in F#?
A tuple is a collection of values and the values may be same or different types.
8. What is list in F#?
List is an immutable series of same type of elements.
It is equivalent to linked list data structure to some extent.
9. What is Enumeration in F#?
Enumerations are also known as enums and enum is a combination of labels and values. In place of literals you can use enums to make code more readable and maintainable.
10. What is delegate in F#?
It is a reference type of variable and it holds the reference of a method.
In F# delegates are similar to pointers.
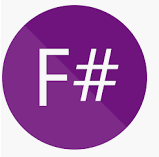
11. What are the types of access control in F#?
There are three types of access control are:
- Public
- Private
- Internal
12. What is Records in F#?
Records: It is used to store any type of data. Here, records are immutable and you can’t change the original records.
13. Can a function return multiple values in F#?
Yes, you can return multiple values in a function by using tuples.
14. What are options in F#?
It is used in calculations when there is a case of exception.
It is also used to indicate whether the function has succeeded or failed.
Option values are of two types:
- Some(x)
- None
15. What are the various ways of creating lists?
Ways of creating lists are:
- By using list literals.
- By using cons operator.
- By using the List.init method.
- By using some syntactic constructs.
16. Who is the developer of F# programming language?
Don Syme is the developer of F# programming language.
17. What are the uses of F# programming language?
The uses of F# programming language are:
- It is used for Financial modeling
- Graphic design
- Compiler programming
- Making scientific model
- Artificial intelligence research work
- Mathematical problem solving
18. Name some reserved keywords available in F#?
Reserved keywords available in F# are:
- abstract and as assert base
- begin do exception extern global
- class done end false if
- default downcast else finally inline
- delegate downto elif function lazy
19. What is the use of delegate keyword in F#?
delegate: It is used to declare a delegate.
20. What is the use of assert keyword in F#?
assert: It is a reserved keyword which is used to verify code during debugging.
21. What is the use of let keyword in F#?
let keyword: This keyword is used for variable declaration in F# programming language.
22. Are variables immutable in F#?
Yes, variables are immutable (once a variable is bound to a value, it can’t be changed.) in F#.
23. Which keyword is used to declare mutable variables in F#?
By using mutable keyword, you can declare mutable variables in F#.
24. What are the types of operators available in F#?
The types of operators available in F# are:
- Boolean Operators
- Bitwise Operators
- Arithmetic Operators
- Comparison Operators
25. What is the use of “<>” operator in F#?
“<>” operator: It is a comparison operator which is used to check if the values of two operands are equal or not. If values are not equal then condition becomes true.
26. What are the ways that makes the compiler ignore the escape sequence?
There are two ways makes the compiler ignore the escape sequence:
- Using the @ symbol
- Enclosing the string in triple quotes
27. What is verbatim string?
verbatim string: A string literal is preceded by the @ symbol, It is known as verbatim string.
28. What are the ways of creating Sets in F#?
The ways of creating Sets in F# are:
- By converting sequences and lists to sets.
- By creating an empty set using Set.empty and adding items using the add function.
29. Which operator is used to access array elements in F#?
dot operator is used to access array elements in F#.
30. What is Array.rev function?
Array.rev function: It is used to generate a new array by reversing the order of an existing array.
31. What is a self in F#?
In F#, a self is used to refer the current object of class type. Self is the same as this keyword in C# and Java. You can name the self-identifier however you want. You are not restricted to names such as this or self as in .Net languages.
Example
type Employee(id,name) as this =
let id = id
let name = name
do this.Display() // This is how we can use self(this) object
member this.Display() =
printf “%d %s” id name
let e =new Employee(100, “Rajkumar”)
32. What is static in F#?
In F#, static is a keyword. It is used to make the static field or static method. Static is not the part of the object. It has its memory space to store static data. It is used to share common properties among objects.
Example
type Account(accno,name) = class
static let rateOfInterest = 8.8
member this.display()=
printfn “%d %s %0.2f” accno name rateOfInterest
end
let a1 = new Account(101,”Rajkumar”)
let a2 = new Account(102, “john”)
a1.display()
a2.display()
33. What is an inheritance in F#?
In F#, inheritance is a process in which child class acquires all the properties and behaviors of its parent class automatically. It is used to reuse the code.
Example
type Employee(name:string) =
class
member this.ShowName() = printf”Name = %s\n” name
end
type Manager(name,salary:int) =
class
inherit Employee(name)
member this.ShowSalary() = printf”Salary = $%d” salary
end
let manager = new Manager(“Rajkumar”,10000)
manager.ShowName()
manager.ShowSalary()
34. What is method overriding in F#?
Method overriding is a feature of Object-oriented programming approach. It helps to achieve polymorphism. We can achieve method overriding using inheritance.
Example
type Employee() =
class
abstract ShowName : unit -> unit
default this.ShowName() = printfn”This is base class method”
end
type Manager() =
class
inherit Employee()
override this.ShowName() = printf “This is derived class method”
end
let employee = new Employee()
let manager = new Manager()
employee.ShowName()
manager.ShowName()
35. What is an abstract class?
Abstract classes are used to provide the full implementation of class members. It may contain non-abstract methods. A class that inherits abstract class must provide an implementation of all abstract methods of the abstract class.
Example
[<AbstractClass>]
type AbstractClass() =
class
abstract member ShowClassName : unit -> unit
end
type DerivedClass() =
class
inherit AbstractClass()
override this.ShowClassName() = printf “This is derived class.”
end
let a = new DerivedClass()
a.ShowClassName()
36. What is an interface in F#?
F# provides Interface type. It provides pure abstraction. It is a collection of abstract methods.
Example
type IEmployee =
abstract member ShowName : unit -> unit
type Manager(id:int, name:string) =
interface IEmployee with
member this.ShowName() = printfn “Id = %d \nName = %s” id name
let manager = new Manager(100,”RajKumar”)
//manager.ShowName() // error: you can’t directly access interface abstract method by using class object.
// so, we need to upcast class type object to interface type by using :> operator.
(manager :> IEmployee).ShowName()
37. What is type extension in F#?
Type extension allows you to add new members to your previously defined object type.
Example
type ClassExtension() =
member this.ShowBefore() = printfn”Class before extension”
// Define type extension.
type ClassExtension with
member this.ShowAfter() = printfn”Class after extension”
let classExtension = new ClassExtension()
classExtension.ShowBefore()
classExtension.ShowAfter()
38. What is a delegate in F#?
In F#, delegates are reference types. It allows us to call the function as an object. It is a feature of this language. It gives an advantage over the other functional programming languages.
Example
type Deligate() =
static member mul(a : int, b : int) = a * b
member x.Mul(a : int, b : int) = a * b
type Multiply = delegate of (int * int) -> int
let getIt (d : Multiply) (a : int) (b: int) =
d.Invoke(a, b)
let d : Multiply = new Multiply( Deligate.mul )
for (a, b) in [(5, 8) ] do
printfn “%d * %d = %d” a b (getIt d a b)
39. What is the object expression in F#?
F# object expression is a special expression. It creates a new instance of anonymous object type which is based on an existing base type, interface, or set of interfaces.
Example
let ex =
{
new System.Exception() with member x.ToString() = “Hello FSharp”
}
printfn “%A” ex
40. What is Exception handling?
Exception handling is a standard mechanism to handle abnormal termination of the program. The Exception is a situation that occurs during program execution. It may lead to terminate program abnormally like divide by zero or a null pointer.
Example
let ExExample a b =
let mutable c = 0
c <- (a/b)
printfn “Rest of the code”
ExExample 10 0
41. What is a try-with block in F#?
In F#, you can create a user-defined exception. It provides flexibility to define custom exceptions according to requirement.
Example
let ExExample a b =
let mutable c = 0
try
c <- (a/b)
with
| 😕 System.DivideByZeroException as e -> printfn “%s” e.Message
printfn “Rest of the code”
ExExample 10 0
42. What is FailWith and InvalidArg in F#?
In F#, you can throw exceptions explicitly. You are allowed to throw a custom exception. You can also throw exceptions by using predefined methods of Exception like Failwith and InvalidArgs.
Example of FailWith keyword
let TestAgeValidation age =
try
if (age<18) then failwith “Sorry, Age must be greater than 18”
with
| Failure(e) -> printfn “%s” e;
printf “Rest of the code”
TestAgeValidation 10
Example of InvalidArg keyword
let TestInvalidArgument age =
if(age<18) then
invalidArg “TestInvalidArgument” (“Sorry, Age must be greater than 18”)
TestInvalidArgument 10
43. What is an assertion in F#?
The assert expression is a debugging feature of F#. You can use it to test an expression. It generates a system error dialog box upon failure in Debug mode.
Example
let divide (x:int, y:int):int =
assert (x>0)
let z = (x/y)
z
let result = divide(10,2)
printf “%d” result
44. What is a module in F#?
The Module is a collection of classes, functions, and types. It helps to organize related code so we can maintain code easily.
Example
open Arithmetic
printf “%d” (sub 20 10)
45. What is the access control in F#?
Access control specifies the accessibility of code. By using these, you can specify the scope of data, method, class, etc.
There are 3 types of access control in F#.
- Public: Public access control also known as default. It is accessible for all. If you don’t specify any access control explicitly in your code, by default it follows public access control.
- Private: F# provides private keyword to declare private members in the class or type. The scope of private is limited to the local block in which it is declared.
- Internal: Internal access control is only accessible from the same assembly. An assembly is a file which is automatically generated by your compiler after compilation of F# code.
46. What is Resource management in F#?
F# manages resources with the help of use and using keywords. Resources may be data, a file or network, etc. It acquires a resource from the operating system or other service providers so that it can be provided to other application.
Example
open System.IO
let ReleaseFile fileName content =
using (System.IO.File.CreateText(fileName)) ( fun textFile ->
textFile.WriteLine(“{0}”, content.ToString() )
)
ReleaseFile “file/textFile.txt” “This is a text file. \nIt contains data.”
47. What are the attributes in F#?
In F#, the attribute is used to enable metadata for a program code construct. The attribute can be applied to any construct like function, module, method, and type.
Example
open System
[<Obsolete(“Do not use. Use newFunction instead.”)>]
let updateSystem() =
printf “updating…”
updateSystem
48. What is a signature in F#?
In F#, the signature file contains information about the public signatures. Signatures can be of a set of program elements, such as types, namespaces, and modules.
Signature file named as signature.fsi
namespace FSharpPrograms
module Arithmetic =
val add : int * int -> int
val sub : int * int -> int
49. What is an open keyword in F#?
An import declaration specifies a module or namespace. You can reference its elements without using a fully qualified name.
Example
open System
Console.WriteLine(“Hello, this is F# here.”)
50. What is the purpose of ‘base’ keyword?
The ‘base’ keyword is used as the name of the base class object.
51. What is the purpose of ‘begin’ keyword?
It is used to signify the starting of a code block.
52. What is the purpose of ‘elif’ keyword?
It is used same as else if branching.
53. What is the purpose of ‘yield’ keyword?
Yield keyword finds its use in the sequence expressions to produce a sequence value.
54. What is the purpose of ‘rec’ keyword?
It is used to indicate a recursive function.
55. What is the purpose of ‘extern’ keyword?
It is used to indicate that the program element declared is defined in some other assembly or binary.
56. Write the syntax for declaration of discriminated unions.
type name =
| case-identifier1 [of [ fieldname1 : ] type1 [ * [ fieldname2 : ]
type2 …]
| case-identifier2 [of [fieldname3 : ]type3 [ * [ fieldname4 : ]type4 …]
57. ‘Variables in F# are immutable’ Explain.
It means, once the value is assigned to a variable it cannot be altered.
58. What is the use of ‘raise’ function?
It is used for the indication of error occurrence.
59. What is lazy computation in F#?
Lazy computation is a feature of F#. Lazy computation does not evaluate immediately. It is executed when the result is needed.
Example
let add x y = x+y
let result = lazy (add 10 10)
printfn “%d” (result.Force())
60. What is XML documentation in F#?
In F#, you can produce documentation from triple-slash (///) code comments. XML comments can precede declarations in code files (.fs) or signature (.fsi) files.
Example
let add x y = x+y
let result = lazy (add 10 10)
printfn “%d” (result.Force())